Extract the ZIP file and look for a file named jscomposer.zip. This is a plugin archive file, which you must upload to your WordPress server. So log in to your WordPress admin panel and go to Plugins Add New. Then click on the Upload Plugin button and select jscomposer.zip. Workspaces in Code Composer Studio contain a lot of metadata. It is possible for this data to get corrupted over time and thus it is a good idea to clean your workspace periodically.
July 19, 2019Louis-Marie Michelin11 min read
I recently started programming in PHP using the well-known Symfony framework. I wanted to keep my VS Code habits and proficiency I had from my previous projects in Node.js and Vue.js, so I tried to configure VS Code for PHP development as well. Moreover, I didn't want to invest €199 in the famous PHPStorm IDE… 😕
The book functions as both a user-empathic exploration of programming and a nitty-gritty JavaScript (ES2020) manual, training the reader to consider how each design decision and language construct might help or hinder the quality of their code and the experiences it creates. Take a look: Clean Code in JavaScript (Publisher Link) Thanks for reading!
TL;DR
VS Code extensions you should install and configure for PHP development:
In this article, I will explain how I managed to make my development environment comparable to PhpStorm's. The commands will be detailed for Ubuntu 18.04, but they can be adapted for Mac OS and possibly for Windows.
I will take an empty Symfony application as an example for this article. Let's start!
Prerequisites
Install PHP and Composer
To be able to run PHP code you obviously need to install PHP. You will also probably need Composer, a commonly used dependency manager for PHP. You can install them by running the following command:
You can install php-all-dev
instead of php-cli
if you want to install some useful libraries as well.
Create an empty Symfony project
Let's now create an empty Symfony app. Then open VS Code and launch the development server:
Increase the number of system watchers if needed
VS Code needs to watch for file changes to work correctly. That's why the following warning may appear because our Symfony project contains a lot of files:
To fix it, you can run the following commands in your terminal, and then restart VS Code:
Go to http://127.0.0.1:8000 from your favorite web browser and check that your Symfony website works.
Autocomplete & Intellisense & Go to definition
Let's create a new route in our application. Just add an empty file HelloWorldController.php
in the src/Controller
folder.
Install PHP Intelephense
By default, VS Code provides some basic code suggestions but they are generic and quite useless. To get useful code suggestions as you type, I recommend installing thePHP Intelephense extensionfor VS Code. I also tried the PHP Intellisense extension but it's slower on big projects and provides fewer features (it doesn't gray out unused imports for example).
Now that the PHP Intelephense extension is installed, we can start writing our HelloWorldController!
As you can see, code suggestions are very relevant and VS Code auto imports the corresponding namespace when you validate a suggestion!
Disable basic suggestions
PHP Intelephense can also auto-suggest relevant methods when typing $myVar->
. The problem is that these suggestions are polluted by the default PHP suggestions.
Basic PHP code suggestions we want to disable
To fix that, you can disable these basic suggestions in VS Code settings. Simply open VS Code's settings.json
file (press Ctrl + ,
then click on the top right {}
icon) and add the following line:
When it's done, you can see useful suggestions!
Relevant suggestions when basic suggestions are disabled
Enable autocomplete in comments / annotations
When you write PHP code, a good practice is to add PHPDoc annotations to make your code more understandable and to help your IDE provide you with relevant code suggestions. By default, VS Code doesn't suggest anything while writing annotations. To activate code suggestions in comments, open the settings.json
file and add the following line:
Code suggestions in annotations
Allow autocomplete in tests
By default, PHP Intelephense excludes Symfony test folders from indexing because the default exclude settings contains a too generic pattern:
To enable suggestions for Symfony test classes, all you need to do is edit your settings.json
file and add:
As you can see, the last line is more specific than in the default settings.
The result 😉:
Code completion enabled for tests
Go to definition
When you want to get more information about a function, you can Ctrl+Click on it and VS Code will open the line where the function is declared!
Generate getters and setters for class properties
If you want VS Code to generate getters and setters for you, you can install this extension. Then, right-click on a class property and select Insert PHP Getter & Setter.
Generate getter and setter
Debugging
Debugging your PHP code can be painful without a debugger. You can use dump($myVar); die();
(😕) but it's not very convenient..
The best way to debug your PHP code is to use Xdebug, a profiler and debugger tool for PHP.
Install Xdebug
Xdebug is not shipped with PHP, you need to install it on your development environment:
Then run sudo nano /etc/php/7.2/mods-available/xdebug.ini
and add the following lines:
Tip! If your PHP project runs in a Docker container, you need also to add the following line to the xdebug.ini
file, where 172.17.0.1
is the IP address of the docker0
interface on your computer (on Mac OS you have to put host.docker.internal
instead):
Check that Xdebug is successfully installed by running php -v
. You should get something like:
Configure VS Code debugger to listen for Xdebug
To be able to use Xdebug in VS Code, you need to install thePHP debug extension.
Then go to the debug tab in VS Code (accessible in the side menu) and click on Add configuration. Select PHP, and close the newly created launch.json
file:
Configure VS Code debugger to listen for Xdebug
Tip! If your PHP project runs in a Docker container, you need to add the following path mapping to the launch.json
file in the Listen for Xdebug
section:
Your debugging environment is now ready! 🕷🚀
Debug your code with VS Code
Let's debug our HelloWorldController.php
to see which are the query parameters given by the user. You can use the following code for example:
Press F5 in VS Code to start the debugging session.
Uncheck the _Everything _option in the breakpoints list and put a breakpoint at line 18 by clicking left of the line number. Then go to http://127.0.0.1:8000/hello?name=Louis. You can now see the value of local variables by hovering the mouse pointer over them:
Request to http://127.0.0.1:8000/hello?name=Louis paused in debugger
You can do a lot of amazing things with the debugger 😃, like:
- adding or removing breakpoints
- stepping over, into or out
- watching variables
- evaluating expressions in the debug console
For more information about the power of VS Code debugger, you can visit the official website.
Configure Xdebug to open file links with VS Code
When you encounter an error, or when you use the Symfony debug toolbar or the profiler, you may want to open the desired file directly in your IDE with the cursor at the corresponding line.
To be able to do that, you need to add the following line to your /etc/php/7.2/mods-available/xdebug.ini
:
Tip! If you run PHP in a Docker container, you can specify a path mapping between your host and your docker like this:
Now, when an error occurs, you can click on it and go directly to the corresponding line in VS Code:
When you visit a route, you can also jump to the corresponding controller by clicking on the debugging toolbar:
Formatting & linting
Formatting and linting your code is a very good practice to keep it clean automatically, and to inform you about some errors.
PHP CS Fixer
The best and commonly used linter for PHP isPHP CS Fixer. It provides a large number of rules to keep your code clean. You can visit this website for more information about the rules and the categories they belong to.
Integrate PHP CS Fixer into VS Code
To be able to auto-format your PHP files in VS Code, you need to install thephp cs fixer extension.
The most complete and safe category of rules is @PhpCsFixer. It's a good point to start. To enable this set of rules in VS Code, open settings.json
and add the following line:
To auto-format your PHP files on save, you also need to add the following lines to your settings.json
:
Auto-format on save with PHP CS Fixer
PHP CS Fixer config file
If you want to disable or enable some specific linting rules, you can do it in a .php_cs
file at the root folder of your project. If this configuration file is present, VS Code takes it into account and overrides the configuration found in settings.json
. You can find more information about the .php_cs
file in the GitHub repository. A simple config file to use @PhpCsFixer category and disable some rules could be:
Bonus: add Prettier to PHP CS Fixer
Prettier for PHP is a code formatter which makes some improvements that PHP CS Fixer does not do. It allows you, among other things, to configure a max line length and makes your code even cleaner.
To add Prettier for PHP to your PHP CS Fixer configuration, you can follow these instructions.
In case of issues with format on save
Depending on your computer's speed, the length of your files and the number of rules you activate, linting a file on save can be slow, causing VS Code to refuse it. To fix this behavior, change the formatOnSaveTimeout in your settings.json
:
Twig support
To activate syntax highlighting for Twig files in VS Code, you need to install theTwig Language 2 extension.
To enable emmet suggestions like in HTML files, add the following line to your settings.json
:
Database management
A good VS Code extension to manage your databases is SQLTools. Feel free to install it and manage your databases directly from your IDE!
Enjoy coding in PHP with VS Code 🚀 and feel free to give me your feedback! 😉
Want to learn more about how Theodo can help bring your Symfony project to the next level?Learn more about our team of Symfony experts. 59: taking the plunge.
Louis-Marie Michelin
Web Developer at Theodo
'Any fool can write code that a computer can understand. Good programmers write code that humans can understand.'
As eloquently noted by Robert Martin in his book “Clean Code,” the only valid measurement of code quality is the number of WTFs per minute as represented in the below diagram:
“Are we debugging in a panic, poring over code that we thought worked? Are customers leaving in droves and managers breathing down our necks? How can we make sure we wind up behind the right door when the going gets tough? The answer is: craftsmanship.”
There are countless examples of bad code bringing companies down or making a disaster of an otherwise good product. Some famous and (fairly serious!) examples include space rockets being mislaunched due to a badly transcribed formula (a single line was missing that meant the rocket had to be terminated 293 seconds after launch ultimately costing around 20 million dollars), or a race condition bug in a medical machine that caused the death of three people, when they were exposed to lethal radiation doses – and you think you’ve had a bad day!
The quality of the code is directly correlated to the maintainability of the product. Programming productivity has an arccotangent relationship with time.
As features are added and changes are made, time passes and the original developers move on or forget some of the project details, or if the quality of the code is not good, changes become increasingly risky and more complex.
Programmers are really authors, and your target audience is not the computer it is other programmers (and yourself). The ratio of time spent by a programmer reading code to writing code is normally 10 to one. You are constantly reading old code in order to write new code.
Writing clean code makes the code easier to understand going forward and is essential for creating a successful maintainable product.
“Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live.”
Remember the second law of thermodynamics? It implies that disorder in a system will always increase unless you spend energy and work to keep it from increasing.
Similarly, it takes a hell of a lot of more effort to write clean code. Writing clean code is hard work. It needs a lot of practice and focus during execution.
To be able to write clean code you should train your mind over a period of time.The hardest part is simply making a start, but as time goes by and your skillset improves, it becomes easier. Writing clean code is all about readability, so even the smallest things like changing you habits for naming variables make the biggest difference.
We should be consciously trying to improve code quality and decrease code entropy. The boy scouts of America have a simple rule:
“Leave the campground cleaner than you found it.”
The same rule applies to programmers. Always leave the checked in code cleaner than the code that is checked out.
Js Composer Wordpress
1. Variable and method name
1.1. Use intention-revealing name
This is bad:
protected $d; // elapsed time in days
This is good:
protected $elapsedTimeInDays;
protected $daysSinceCreation;
protected $daysSinceModification;
protected $fileAgeInDays;
1.2. Use pronounceable name
This is bad:
public $genymdhms;
public $modymdhms;
This is good:
public $generationTimestamp;
public $modificationTimestamp;
1.3. Use namespaces instead of prefixing names
Most modern programming languages (including PHP and Python) support namespaces.
This is bad:
class Part {
private $m_dsc;
}
This is good:
class Part {
private $description;
}
Inpixio download for mac.
1.4. Don't be cute
This is bad:
$program->whack();
This is good:
$program->kill();
1.5. Use one word per concept
Be consistent. For example, don’t use getand fetchto do the same thing in different classes
1.6. Use solution domain names
People reading your code will be other programmers so they understand solution domain terminology, so make the most of it. - For example, jobQueue is better than jobs
1.7. Use verbs for function names and nouns for classes and attributes
class Product {
private $price;
public function increasePrice($dollarsToAddToPrice)
{
$this->price += $dollarsToAddToPrice;
}
}
2. Better Functions
2.1. The smaller the better
How To Clean Code Js Composer Free
2.2. A function should only do one thing
2.3. No nested control structure
2.4. Less arguments are better
More than three arguments are evil. For example:
Circle makeCircle(Point center, double radius);
Is better than
Circle makeCircle(double x, double y, double radius);
2.5. No side effects
Functions must only do what the name suggests and nothing else.
2.6. Avoid output arguments
If returning something is not enough then your function is probably doing more than one thing.
For example
email.addSignature();
Is better than
addSignature(email);
2.7. Error Handling is one thing
Throwing exceptions is better than returning different codes dependent on errors.
Asking for forgiveness is easier than requesting permission. Use try/catch instead of conditions if possible
2.8. Don’t repeat yourself
Functions must be atomic
3. Comments
3.1. Don’t comment bad code, rewrite it
3.2. If code is readable you don’t need comments
This is bad:
// Check to see if the employee is eligible for full benefits
if ($employee->flags && self::HOURLY_FLAG && $employee->age > 65)
This is good:
if ($employee->isEligibleForFullBenefits())
3.3. Explain your intention in comments
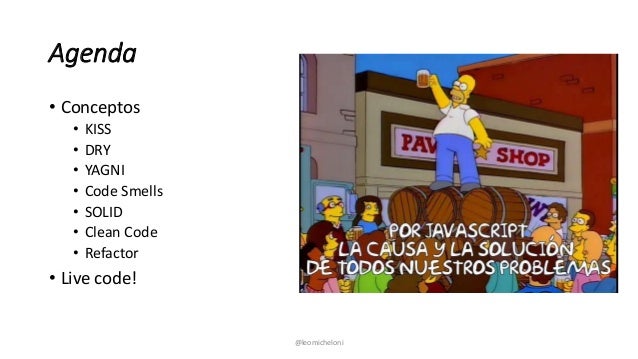
For example:
// if we sort the array here the logic becomes simpler in calculatePayment() method
3.4. Warn of consequences in comments
For example:
// this script will take a very long time to run
3.5. Emphasis important points in comments
For example:
// the trim function is very important, in most cases the username has a trailing space
3.6. Always have your PHPDoc comments
Most IDEs do this automatically, just select the shortcut.
Having doc comments are especially important in PHP because methods don’t have argument and return types. Having doc comments lets us specify argument and return types for functions. Can i download google calendar on mac.
If your function name is clear, you don’t need a description of what the method is doing in the doc comment.
For example:
/**
* Return a customer based on id
* @param int $id
* @return Customer
*/
Public function getCustomerById($id)
3.7. Any comments other than the above should be avoided
3.8. Noise comments are bad
For example:
/** The day of the month. */
private $dayOfMonth;
3.9. Never leave code commented
Perhaps this is the most important point in this whole article. With modern source control software such as Git you can always investigate and revert back to historical code. It is ok to comment code when debugging or programming, but you should never ever check in commented code.
How To Clean Code Js Composer Tutorial
4. Other code smells and heuristics
There are a lot more that you can do to identify and avoid bad code. Below is a list of some code smells and anti-patterns to avoid. Refer to the sources of this blog for more information.
- Dead code
- Speculative Generality - no need “what if?”
- Large classes
- God object
- Multiple languages in one file
- Framework core modifications
- Overuse of static (especially when coding in Joomla!)
- Magic numbers - replace with const or var
- Long if conditions - replace with function
- Call super’s overwritten methods
- Circular dependency
- Circular references
- Sequential coupling
- Hard-coding
- Too much inheritance - composition is better than inheritance
Conclusion
As I mentioned before it takes a lot of practice and effort to write clean code. However it is definitely worth the extra effort. Writing clean code is integral to the success of the project.
Adopting coding standards such as (PSR-2) will make it a lot easier to write clean code. If you are using PhpStorm (IntelliJ) you can set your coding style to PSR-2 and the IDE’s internal code inspector will warn you when your code is not good.
Also adopting Code Sniffer will help a lot. You can install Joomla’s CodeSniffer and only commit when it is green.
Perfect code is not always feasible but It is important to try and write code as cleanly as possible. Programming is an art. Let other programmers enjoy your code.
To finish, I would like to share a telling quote from Robert Martin:
“[Most managers] may defend the schedule and requirements with passion; but that’s their job. It’s your job to defend the code with equal passion”
Sources
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
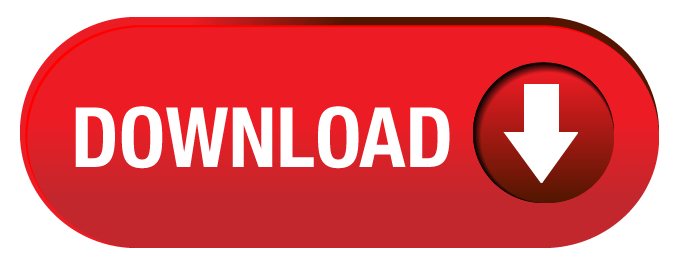